很明显Flask是一个轻量级框架
安装Flask
进入D:\Python\Scripts输入
第一个Hello World
创建一个demo0.py文件
1 2 3 4 5 6 7 8 9 10
| from flask import Flask
app = Flask(__name__)
@app.route('/') def hello_world(): return 'Hello World'
if __name__ == '__main__': app.run()
|
运行
好的, 第一个Hello World就成功运行起来了
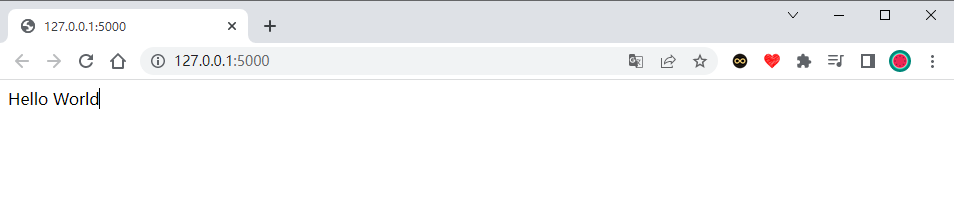
接下来手搓一个后台管理系统吧^_^
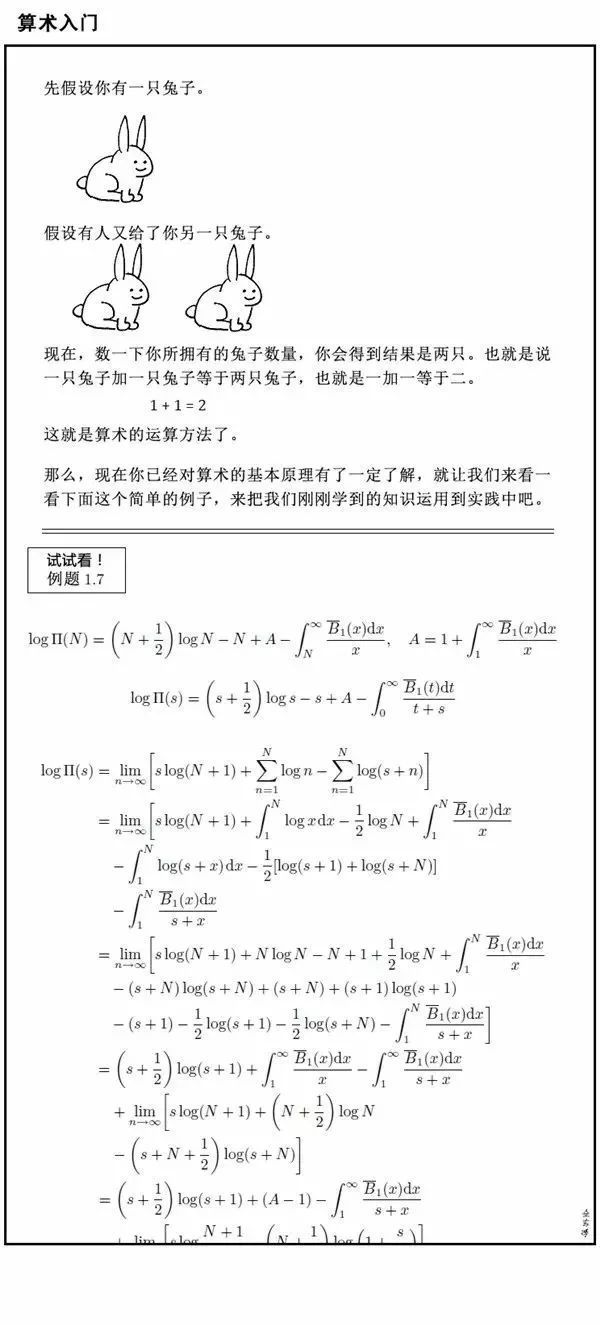
run()函数
1
| app.run(host, port, debug, options)
|
host就是主机名, 默认127.0.0.1, 设置成0.0.0.0可以暴露给其他主机访问
port就是端口
debug表示调试模式, 在程序运行时, 如果修改了代码, 调试模式就类似于热部署了
options表示要转发给底层的服务器
路由
@app.route后面就是访问的路径
http://127.0.0.1:666/hello
1 2 3
| @app.route('/hello') def hello_world(): return 'hello world'
|
也可以改写成如下的形式
1 2 3 4 5
| def hello_world(): return 'Hello World!!'
app.add_url_rule('/hello', 'hello', hello_world)
|
app.add_url_rule用来绑定URL和函数, 中间的参数是路由的端点, 唯一标识路由的字符串
接收参数
1 2 3
| @app.route('/hello/<name>') def hello_world(name): return 'Hello%s World!!' % name
|
路由上定义一个参数, 然后函数上接收这个参数, 最后将这个参数返回给页面

一般来说默认是字符串类型的, 当然也可以指定类型
比如int, float
1 2 3 4 5 6 7 8 9
| @app.route('/num/<int:num>') def get_num(num): return '%d' % num
@app.route('/float/<float:num>') def get_float(num): return '%f' % num
|
http://127.0.0.1:666/num/1
http://127.0.0.1:666/float/1.0
1 2 3 4 5 6 7
| @app.route('/flask') def hello_flask(): return 'Hello Flask'
@app.route('/python/') def hello_python(): return 'Hello Python'
|
http://127.0.0.1:666/flask 可以正常请求
http://127.0.0.1:666/flask/ 不可以正常请求
http://127.0.0.1:666/python 正常请求
http://127.0.0.1:666/python/ 正常请求
url_for
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
|
from flask import Flask, redirect, url_for app = Flask(__name__) @app.route('/admin') def hello_admin(): return 'Hello Admin'
@app.route('/guest/<guest>')
def hello_guest(guest): return 'Hello %s as Guest' % guest
@app.route('/user/<name>') def hello_user(name): if name =='admin': return redirect(url_for('hello_admin')) else: return redirect(url_for('hello_guest', guest = name))
if __name__ == '__main__': app.run(debug = True)
|
url_for是用来生成url的
url_for(‘hello_admin’) => /user/admin => /admin
url_for(‘hello_guest’, guest = name) => /user/123 => /guest/123
当请求的是 http://127.0.0.1:5000/user/123 => http://127.0.0.1:5000/guest/12
返回的Hello 12 as Guest
请求http://127.0.0.1:5000/admin 返回Hello Admin
HTTP方法
创建一个html页面
1 2 3 4 5 6 7 8 9
| <html> <body> <form action = "http://localhost:5000/login" method = "post"> <p>Enter Name:</p> <p><input type = "text" name = "nm" /></p> <p><input type = "submit" value = "submit" /></p> </form> </body> </html>
|
然后http-server运行这个页面
1
| http-server -o .\login.html
|
然后编写如下Python代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| from flask import Flask, redirect, url_for, request, render_template
app = Flask(__name__)
@app.route('/') def index(): return render_template("login.html")
@app.route('/success/<name>') def success(name): return 'welcome %s' % name
@app.route('/login',methods = ['POST', 'GET']) def login(): if request.method == 'POST': print(1) user = request.form['nm'] return redirect(url_for('success',name = user)) else: print(2) user = request.args.get('nm') return redirect(url_for('success',name = user))
if __name__ == '__main__': app.run()
|
当method为post时, 控制台输出的是1
当method为get时, 控制台输出的是2
1
| <form action = "http://localhost:5000/login" method = "post">
|
模板引擎
Flask 是使用 Jinja2 这个模板引擎来渲染模板
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> 我的模板html内容 </body> </html>
|
1 2 3 4 5 6 7
| from flask import Flask, render_template
app = Flask(__name__)
@app.route('/') def index(): return render_template('hello.html')
|
当有人请求 http://localhost:5000/ 将会呈现我的模板html内容